Sum Of Digits | Write a program in Java to print the sum of digits of a number. | ISC Computer Science | ICSE Computer Application
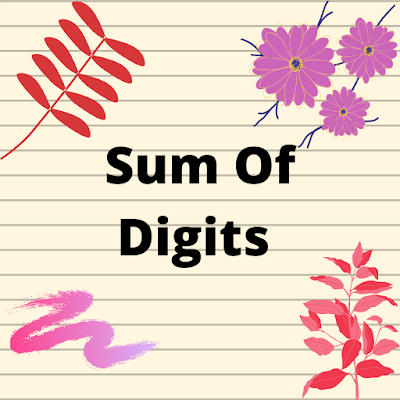
Algorithm
- Start
- in:=0
- temp:=0
- digit:=0
- sum:=0
- Print "Enter a number"
- Read n
- temp=in
- sum=0
- If temp>0, go to step 11 or else go to step 11 or else go to step 15
- digit=temp%10
- sum=sum+digit
- temp=temp/10
- Go to step 10
- Print "The sum of digits of "+in+" is "+sum
- Stop
Program
BufferedReader
import java.io.*;
class DigiSum
{
int in, temp, digit, sum;
void accept() throws IOException
{
BufferedReader br=new BufferedReader (new InputStreamReader (System.in));
System.out.println("Enter a number");
in=Integer.parseInt(br.readLine());
}
void sumOfDigits()
{
temp=in;
sum=0;
while(temp>0)
{
digit=temp%10;
sum=sum+digit;
temp=temp/10;
}
}
void display()
{
System.out.println("The sum of digits of "+in+" is "+sum);
}
void main() throws IOException
{
accept();
sumOfDigits();
display();
}
}
Scanner
import java.util.*;
class DigiSum
{
int in, temp, digit, sum;
void accept()
{
Scanner sc=new Scanner (System.in);
System.out.println("Enter a number");
in=sc.nextInt();
}
void sumOfDigits()
{
temp=in;
sum=0;
while(temp>0)
{
digit=temp%10;
sum=sum+digit;
temp=temp/10;
}
}
void display()
{
System.out.println("The sum of digits of "+in+" is "+sum);
}
void main()
{
accept();
sumOfDigits();
display();
}
}
Documentation
Variable Description
Data Type | Variable Name | Description |
int | in | To store the number accepted by the user |
int | temp | To act as a temp variable |
int | digit | To store the digit of the number |
int | sum | To store the sum of digits of a number |
Method Description
Return Type | Method Name | Description |
void | accept() | To accept data from the user |
void | sumOfDigits() | To calculate the sum of digits of a number |
void | display() | To display the sum of digits |
void | main() | To act as an entry gateway for the compiler |
Output
Share with all ISC Computer Science Students you know.
Comment below with all your program requests and blog suggestions.
Follow us on social media by clicking on these links.
Instagram : @ISCJavaCode
Twitter: @ISCJavaCode
Quora: ISC Java Code
Reddit: @ISCJavaCode
Stay Healthy
Stay Happy
Stay Helpful
Comments
Post a Comment