Insertion Sort | Write a program in java to perform insertion sort on an array. | ISC Computer Science | ICSE Computer Applications
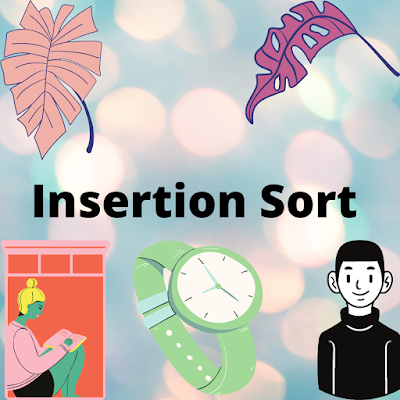
Algorithm
- Start
- a:=null
- i:=0
- n:=0
- j:=0
- temp:=0
- Print "Enter the size of the array"
- Read n
- a=new int[n]
- i=0
- If i<n, go to step 12 or else go to step 15
- Print "Enter a number"
- Read a[i]
- i++. Go to step 11
- i=0
- If i<n, go to step 17 or else go to step 25
- temp=a[i]
- j=i-1
- If j>=0 && a[j]>temp, go to step 20 or else go to step 23
- a[j+1]=[j]
- j=j-1
- Go to step 19
- a[j+1]=temp
- i++. Go to step 16
- i=0
- If i<n, go to step 27 or else go to step 29
- Print a[i];
- i++. Go to step 26
- Stop
Program
Buffered Reader
import java.io.*;
class SortArray
{
int a[], i, n, j, temp;
void accept() throws IOException
{
BufferedReader br=new BufferedReader(new InputStreamReader (System.in));
System.out.println("Enter the size of the array");
n=Integer.parseInt(br.readLine());
a=new int[n];
for(i=0;i<n;i++)
{
System.out.println("Enter a number");
a[i]=Integer.parseInt(br.readLine());
}
}
void sort()
{
for(i=0;i<n;i++)
{
temp=a[i];
j=i-1;
while(j>=0&&a[j]>temp)
{
a[j+1]=a[j];
j=j-1;
}
a[j+1]=temp;
}
}
void display()
{
for(i=0;i<n;i++)
{
System.out.println(a[i]);
}
}
void main() throws IOException
{
accept();
sort();
display();
}
}
Scanner
import java.util.*;
class SortArray
{
int a[], i, n, j, temp;
void accept()
{
Scanner sc=new Scanner(System.in);
System.out.println("Enter the size of the array");
n=sc.nextInt();
a=new int[n];
for(i=0;i<n;i++)
{
System.out.println("Enter a number");
a[i]=sc.nextInt();
}
}
void sort()
{
for(i=0;i<n;i++)
{
temp=a[i];
j=i-1;
while(j>=0&&a[j]>temp)
{
a[j+1]=a[j];
j=j-1;
}
a[j+1]=temp;
}
}
void display()
{
for(i=0;i<n;i++)
{
System.out.println(a[i]);
}
}
void main()
{
accept();
sort();
display();
}
}
Documentation
Variable Description
Data Type | Variable Name | Description |
int[] | a | To store an array of numbers |
int | n | To store the size of the array |
int | i | To act as counter variable |
int | j | To act as a counter variable |
int | temp | To act as a temp variable |
Method Description
Return Type | Method Name | Description |
void | accept() | To accept data from the user |
void | sort() | To sort data in the array using insertion sort |
void | display() | To display the array |
void | main() | To act as an entry gateway for the compiler |
Output
Share with all ISC Computer Science Students you know.
Comment below with all your program requests and blog suggestions.
Follow us on social media by clicking on these links.
Instagram : @ISCJavaCode
Twitter: @ISCJavaCode
Quora: ISC Java Code
Reddit: @ISCJavaCode
Stay Healthy
Stay Happy
Stay Helpful
Comments
Post a Comment