Sum of Two Arrays | Write a program in Java to find the sum of two arrays. | ISC Computer Science | ICSE Computer Applications |
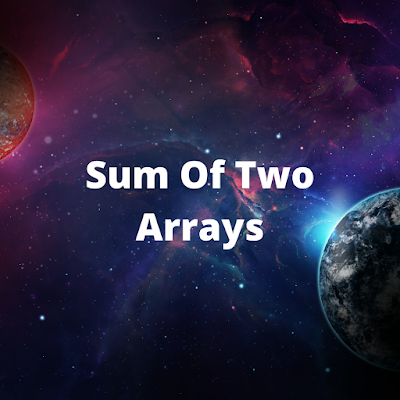
Algorithm
- Start
- a[]=null
- b[]=null
- sum[]=null
- i:=0
- n:=0
- Print "Enter the length of both the array"
- Read n
- a=new int[n]
- b=new int[n]
- sum=new int[n]
- i=0
- If i<n, go to step 14 or else go to step 19
- Print "Enter a number in the first array"
- Read a[i]
- Print "Enter a number in the second array"
- Read b[i]
- i++. Go to step 13
- i=0
- If i<n, go to step 21 or else go to step 23
- sum[i]=a[i]+b[i]
- i++. Go to step 20
- i=0
- If i<n, go to step 25 or else go to step 27
- Print sum[i]
- i++. Go to step 24
- Stop
Program
Buffered Reader
import java.io.*;
class SumArray
{
int a[], b[], sum[], i, n;
void accept() throws IOException
{
BufferedReader br=new BufferedReader (new InputStreamReader (System.in));
System.out.println("Enter the length of both the array");
n=Integer.parseInt(br.readLine());
a=new int[n];
b=new int[n];
sum=new int[n];
for(i=0;i<n;i++)
{
System.out.println("Enter a number in the first array");
a[i]=Integer.parseInt(br.readLine());
System.out.println("Enter a number in the second array");
b[i]=Integer.parseInt(br.readLine());
}
}
void add()
{
for(i=0;i<n;i++)
{
sum[i]=a[i]+b[i];
}
}
void display()
{
for(i=0;i<n;i++)
{
System.out.println(sum[i]);
}
}
void main() throws IOException
{
accept();
add();
display();
}
}
Scanner
import java.util.*;
class SumArray
{
int a[], b[], sum[], i, n;
void accept()
{
Scanner sc=new Scanner(System.in);
System.out.println("Enter the length of both the array");
n=sc.nextInt();
a=new int[n];
b=new int[n];
sum=new int[n];
for(i=0;i<n;i++)
{
System.out.println("Enter a number in the first array");
a[i]=sc.nextInt();
System.out.println("Enter a number in the second array");
b[i]=sc.nextInt();
}
}
void add()
{
for(i=0;i<n;i++)
{
sum[i]=a[i]+b[i];
}
}
void display()
{
for(i=0;i<n;i++)
{
System.out.println(sum[i]);
}
}
void main()
{
accept();
add();
display();
}
}
Documentation
Variable Description
Data Type | Variable Name | Description |
int[] | a | To store the first array |
int[] | b | To store the second array |
int[] | sum | To store the sum of the two arrays |
int | n | To store the length of the arrays |
int | i | To act as a counter variable |
Method Description
Return Type | Method Name | Description |
void | accept() | To accept data from the user |
void | add() | To find the sum of the two arrays |
void | display() | To display the sum of the array |
void | main() | To act as an entry gateway for the compiler. |
Output
Share with all ISC Computer Science Students you know.
Comment below with all your program requests and blog suggestions.
Follow us on social media by clicking on these links.
Instagram : @ISCJavaCode
Twitter: @ISCJavaCode
Quora: ISC Java Code
Reddit: @ISCJavaCode
Stay Healthy
Stay Happy
Stay Helpful
Comments
Post a Comment