Selection Sort An Array | Write a program in Java to selection sort an array. | ISC Computer Science | ICSE Computer Applications
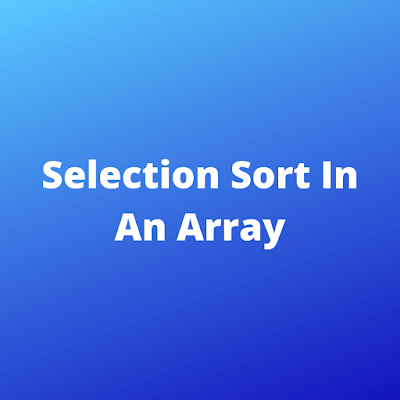
Algorithm
- Start
- a[]=null
- i:=0
- n:=0
- j:=0
- small:=0
- Print "Enter the size of the array"
- Read n
- a=new int[n]
- i=0
- If i<n, go to step 12 or else go to step 15
- Print "Enter a number"
- Read a[i]
- i++. Go to step 11
- i=0
- If i<n, go to step 17 or else go to step 26
- small=a[i]
- j=i
- If j<n, go to step 20 or else go to step 24
- If small>a[j], go to step 21 or else go to step 23
- small=a[j]
- a[j]=a[i]
- j++. Go to step 19
- a[i]=small
- i++. Go to step 16
- i=0
- If i<n, go to step 28 or else go to step 30
- Print a[i]
- i++. Go to step 27
- Stop
Program
Buffered Reader
import java.io.*;
class SortArray
{
int a[], i, n, j, small;
void accept() throws IOException
{
BufferedReader br=new BufferedReader(new InputStreamReader (System.in));
System.out.println("Enter the size of the array");
n=Integer.parseInt(br.readLine());
a=new int[n];
for(i=0;i<n;i++)
{
System.out.println("Enter a number");
a[i]=Integer.parseInt(br.readLine());
}
}
void sort()
{
for(i=0;i<n;i++)
{
small=a[i];
for(j=i;j<n;j++)
{
if(small>a[j])
{
small=a[j];
a[j]=a[i];
}
}
a[i]=small;
}
}
void display()
{
for(i=0;i<n;i++)
{
System.out.println(a[i]);
}
}
void main() throws IOException
{
accept();
sort();
display();
}
}
Scanner
import java.util.*;
class SortArray
{
int a[], i, n, j, small;
void accept()
{
Scanner sc=new Scanner (System.in);
System.out.println("Enter the size of the array");
n=sc.nextInt();
a=new int[n];
for(i=0;i<n;i++)
{
System.out.println("Enter a number");
a[i]=sc.nextInt();
}
}
void sort()
{
for(i=0;i<n;i++)
{
small=a[i];
for(j=i;j<n;j++)
{
if(small>a[j])
{
small=a[j];
a[j]=a[i];
}
}
a[i]=small;
}
}
void display()
{
for(i=0;i<n;i++)
{
System.out.println(a[i]);
}
}
void main()
{
accept();
sort();
display();
}
}
Documentation
Variable Description
Data Type | Variable Name | Description |
int[] | a | To store an array of numbers |
int | n | To store the size of the array |
int | i | To act as a counter variable |
int | j | To act as a counter variable |
int | small | To store the smallest variable |
Method Description
Return Type | Method Name | Description |
void | accept() | To accept data from the user |
void | sort() | To sort data in the array |
void | display() | To display the array |
void | main() | To act as an entry gateway for the compiler |
Output
Share with all ISC Computer Science Students you know.
Comment below with all your program requests and blog suggestions.
Follow us on social media by clicking on these links.
Instagram : @ISCJavaCode
Twitter: @ISCJavaCode
Quora: ISC Java Code
Reddit: @ISCJavaCode
Stay Healthy
Stay Happy
Stay Helpful
Comments
Post a Comment